Why do some stocks have no options? Understand the reasons behind it
Why are some stocks not available for trading? Options trading is a popular investment strategy that allows traders to speculate on the future price …
Read ArticleMoving averages are widely used in financial analysis, signal processing, and data smoothing. They help to identify trends and patterns in data by calculating an average value over a specific period of time. In C programming, calculating moving averages can be done efficiently using arrays and loops.
To calculate a moving average, you need to define the number of values to include in the average (the window size) and create an array to store the data. As new data becomes available, you add it to the array and remove the oldest data point. Then, you calculate the average of the values in the array.
An efficient way to calculate a moving average is to use a sliding window approach. This means that you only need to update the sum of the values in the window instead of recalculating the sum from scratch for each new data point. By keeping track of the sum and the number of values in the window, you can easily calculate the average.
Implementing a moving average algorithm in C involves using loops to iterate through the array and keep track of the sum and the number of values. You can use conditional statements to handle cases where the window is not fully filled yet. Additionally, you can use modular arithmetic to handle cases where the window wraps around to the beginning of the array.
Example:
Let’s say we want to calculate a 5-day simple moving average for a stock price. We have an array of 10 stock prices, and our window size is 5. We start by summing up the first 5 prices and dividing the sum by 5 to get the average. Then, we slide the window one day and repeat the process until we reach the end of the array.
Calculating moving averages in C is a fundamental skill for data analysis and signal processing. By understanding the concept and implementing the algorithm efficiently, you can leverage moving averages to extract valuable insights from your data.
The moving average is a popular statistical calculation technique used to analyze data over a certain period of time. It is commonly used in various fields such as finance, stock market analysis, and time series forecasting. The purpose of calculating the moving average is to smooth out fluctuating data points and identify underlying trends or patterns.
The moving average is calculated by taking the average of a specific number of data points in a defined time period. This time period is commonly referred to as the “window” or “lookback” period. The number of data points included in the calculation and the length of the time period can vary depending on the application and the desired level of smoothing.
The moving average is useful for eliminating random noise and highlighting the overall direction of the data set. It provides a simple way to visualize and interpret trends, reversals, and changes in data over time. By calculating the moving average, analysts and traders can make informed decisions based on historical data and potentially predict future movements in the data.
There are different types of moving averages, including the simple moving average (SMA) and the exponential moving average (EMA). The SMA calculates the average of the data points using equal weights, while the EMA assigns different weights to each data point, giving more importance to recent values. The choice of moving average type depends on the specific requirements of the analysis and the level of sensitivity to recent data.
Read Also: Top Brokers Supporting MetaTrader 4 | Choose the Best for Your Trading Needs
Key takeaways:
Overall, the moving average is a valuable tool in data analysis and forecasting, providing insights into the overall direction and behavior of a dataset over time.
The moving average is an important tool in statistical analysis and time series forecasting. It is used to smooth out fluctuations in data by averaging out the values over a certain period of time. This technique is particularly useful in financial markets, where it helps analysts identify trends and make informed decisions based on historical data.
One of the key advantages of using moving averages is that they provide a clearer picture of the underlying trend in a dataset by removing noise and short-term fluctuations. This can be especially beneficial when analyzing volatile or noisy data, as it helps to filter out random variations and highlight the overall direction of the data.
In addition to trend analysis, moving averages can also be used to generate trading signals. Traders often use moving averages to identify potential buy or sell signals when the price of an asset crosses above or below a moving average line. This can help them to time their trades and take advantage of potential market movements.
Another important application of moving averages is in forecasting future trends. By calculating and analyzing different moving averages, analysts can make predictions about future price movements, sales figures, or any other data series. This can be extremely valuable in making informed decisions and planning for the future.
Overall, moving averages are an essential tool for data analysis, trend identification, and forecasting. They help to filter out noise, identify trends, generate trading signals, and make predictions about future data points. Whether you are a financial analyst, a trader, or a data scientist, understanding and using moving averages can greatly enhance your analytical capabilities.
Calculating the moving average is a common task in data analysis and time series forecasting. It is a statistical indicator that helps smooth out the fluctuations in a dataset by averaging a specified number of consecutive values. In this article, we will learn how to calculate the moving average in C language.
Read Also: Understanding Exotic Options: A Comprehensive Guide
To calculate the moving average, we need a dataset and a window size. The dataset can be an array or a list of numbers, and the window size represents the number of consecutive values to be averaged. The moving average is obtained by summing the values in the window and dividing by the window size.
Here is an example code in C that demonstrates the calculation of the moving average:
[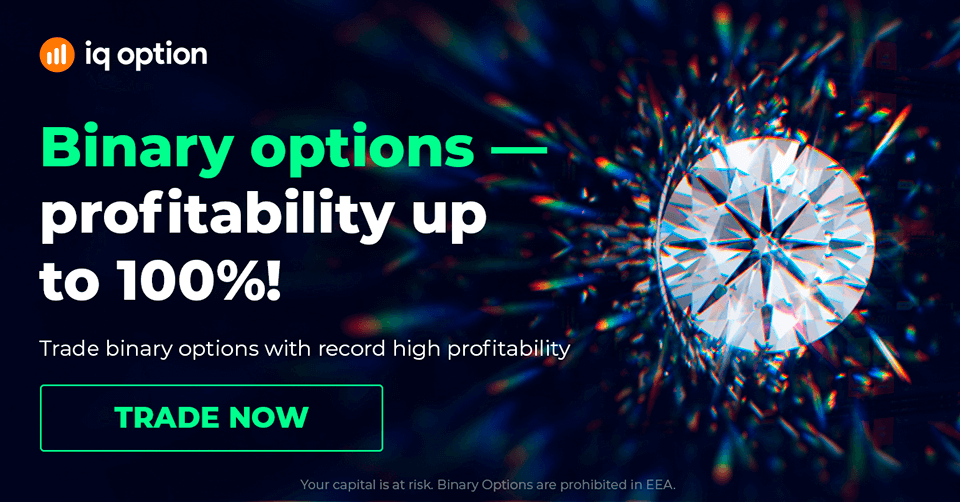](/iq/)
include void calculateMovingAverage(int dataset[], int dataSize, int windowSize){// Iterate through the datasetfor (int i = 0; i <= dataSize - windowSize; i++){int sum = 0;// Calculate sum of values in the windowfor (int j = i; j < i + windowSize; j++){sum += dataset[j];}// Calculate and print the moving averagefloat movingAverage = (float)sum / windowSize;printf("Moving average at index %d: %.2f", i, movingAverage);}}int main(){// Sample datasetint dataset[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};int dataSize = sizeof(dataset) / sizeof(dataset[0]);// Define the window sizeint windowSize = 3;// Calculate the moving averagecalculateMovingAverage(dataset, dataSize, windowSize);return 0;}
=================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================================
In this example, we have a dataset consisting of values from 1 to 10. The window size is set to 3, which means we will average 3 consecutive values. ThecalculateMovingAverage
function takes the dataset, the size of the dataset, and the window size as parameters. It iterates through the dataset and calculates the sum of values in the window using a nested loop. Finally, it calculates the moving average by dividing the sum by the window size and prints the result.
When you run the code, you will get the moving average for each window starting from index 0:
Moving average at index 0: 2.00Moving average at index 1: 3.00Moving average at index 2: 4.00Moving average at index 3: 5.00Moving average at index 4: 6.00Moving average at index 5: 7.00Moving average at index 6: 8.00
This is how you can calculate the moving average in C language. By adjusting the window size, you can control the level of smoothing in the dataset. The moving average is a useful tool for analyzing trends and identifying patterns in time series data.
Moving average is a statistical calculation that is commonly used in finance and data analysis to analyze trends over time.
Moving average is important because it helps to smooth out short-term fluctuations in data and identify long-term trends.
Moving average is calculated by taking the average of a set of data points over a specified period of time, and then moving that average forward in time.
Some common applications of moving average include stock market analysis, weather forecasting, and sales forecasting.
Why are some stocks not available for trading? Options trading is a popular investment strategy that allows traders to speculate on the future price …
Read ArticleIs algorithmic trading the same as systematic trading? Algorithmic trading and systematic trading are two terms that are often used interchangeably in …
Read ArticleWhere can I find a valid promo code? In today’s fast-paced world, everyone loves a good deal. Whether you’re shopping online or in-store, finding a …
Read ArticleExchange Fee for XTB: All You Need to Know When it comes to trading on the XTB platform, understanding the exchange fee is crucial. This fee, also …
Read ArticleHow often are premiums paid on options? An option premium is the price that an investor pays to purchase an options contract. It is a key component in …
Read ArticleCalculating Option Price: How to Determine the Value of an Option Options are a popular financial instrument that give investors the opportunity to …
Read Article